Exception & Assertion
EXCEPTION
- Represents an error condition that can occur during the normal course of program execution
- exception handling increase robustness
EXCEPTION HANDLING
- When an exception occurs -> the normal sequence of flow is terminated and the exception-handling routine is executed
- Exception occurs -> exeption is thrown
- Exception-handling code is executed -> exception is caught
try {
// Block of code to try
}
catch(Exception e){
// Block of code to handle errors
}
TYPE OF EXCEPTIONS
- Checked :
- checked at compile time
- Unchecked / runtime exceptions :
- unchecked at compile time and are detected only at runtime
ASSERTIONS
- A language feature we use to detect logical errors in a program
- display logical error when compilling
TYPE OF ASSERTION
- Postconditon assertion :
- checks for a condition that must be true after a method is executed
</ul>
<li>Precondition assertion :</li>
<ul>
<li>a checking of condition that must be true before a method is executed</li>
</ul>
<li>Control flow invariant</li>
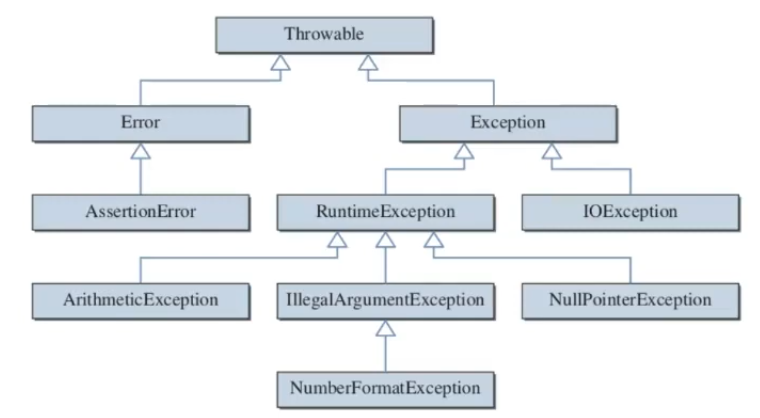
SOURCE CODE :
Execption :
package oop.exceptions;
import java.util.Calendar;
import java.util.GregorianCalendar;
import java.util.Scanner;
// program mencari tahun lahir berdasarkan usia
public class AgeInput1 {
public int getAge(String prompt, Scanner scanner) {
System.out.print(prompt);
int age = scanner.nextInt();
return age;
}
public static void main(String[] args) {
GregorianCalendar today;
int age, thisYear, bornYr;
String answer;
Scanner scanner = new Scanner(System.in);
AgeInput1 input = new AgeInput1();
age = input.getAge("How old are you? ", scanner);
today = new GregorianCalendar();
thisYear = today.get(Calendar.YEAR);
bornYr = thisYear - age;
System.out.print("Already had your birthday this year? (Y or N)");
answer = scanner.next();
if (answer.equals("N") || answer.equals("n")) {
bornYr--;
}
System.out.println("\nYou are born in " + bornYr);
}
}
package oop.exceptions;
import java.util.Calendar;
import java.util.GregorianCalendar;
import java.util.InputMismatchException;
import java.util.Scanner;
public class AgeInput2 {
public int getAge(String prompt, Scanner scanner) {
int age = 0;
boolean keepGoing = true;
while (keepGoing) {
System.out.print(prompt);
try {
age = scanner.nextInt();
keepGoing = false;
} catch (InputMismatchException e) {
scanner.next(); // remove the leftover garbage
// from the input buffer
System.out.println("Invalid Entry.Please enter digits only.");
}
}
return age;
}
public static void main(String[] args) {
GregorianCalendar today;
int age, thisYear, bornYr;
String answer;
Scanner scanner = new Scanner(System.in);
AgeInput2 input = new AgeInput2();
age = input.getAge("How old are you? ", scanner);
today = new GregorianCalendar();
thisYear = today.get(Calendar.YEAR);
bornYr = thisYear - age;
System.out.print("Already had your birthday this year? (Y or N)");
answer = scanner.next();
if (answer.equals("N") || answer.equals("n")) {
bornYr--;
}
System.out.println("\nYou are born in " + bornYr);
}
}
package oop.exceptions;
import java.util.Calendar;
import java.util.GregorianCalendar;
import java.util.InputMismatchException;
import java.util.Scanner;
public class AgeInput3 {
public int getAge(String prompt, Scanner scanner) {
int age = 0;
while (true) {
System.out.print(prompt);
try {
age = scanner.nextInt();
if (age < 0) {
throw new Exception("Negative age is invalid");
}
return age;
} catch (InputMismatchException e) {
scanner.next(); // remove the leftover garbage
// from the input buffer
System.out.println("Invalid Entry.Please enter digits only.");
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
public static void main(String[] args) {
GregorianCalendar today;
int age, thisYear, bornYr;
String answer;
Scanner scanner = new Scanner(System.in);
AgeInput3 input = new AgeInput3();
age = input.getAge("How old are you? ", scanner);
today = new GregorianCalendar();
thisYear = today.get(Calendar.YEAR);
bornYr = thisYear - age;
System.out.print("Already had your birthday this year? (Y or N)");
answer = scanner.next();
if (answer.equals("N") || answer.equals("n")) {
bornYr--;
}
System.out.println("\nYou are born in " + bornYr);
}
}
package oop.exceptions;
import java.util.InputMismatchException;
import java.util.Scanner;
public class AgeInput4 {
private static final String DEFAULT_MESSAGE = "Your age:";
private static final int DEFAULT_LOWER_BOUND = 0;
private static final int DEFAULT_UPPER_BOUND = 99;
private int lowerBound;
private int upperBound;
private Scanner scanner;
public AgeInput4() {
init(DEFAULT_LOWER_BOUND, DEFAULT_UPPER_BOUND);
}
public AgeInput4(int low, int high) throws IllegalArgumentException {
if (low > high) {
throw new IllegalArgumentException("Low (" + low + ") was " + "larger than high(" + high + ")");
} else {
init(low, high);
}
}
public int getAge() throws Exception {
return getAge(DEFAULT_MESSAGE);
}
public int getAge(String prompt) throws Exception {
int age;
while (true) {
System.out.print(prompt);
try {
age = scanner.nextInt();
if (age < lowerBound || age > upperBound) {
throw new Exception("Input out of bound");
}
return age; // input okay so return the value & exit
} catch (InputMismatchException e) {
scanner.next();
System.out.println("Input is invalid.\n" + "Please enter digits only");
}
}
}
private void init(int low, int high) {
lowerBound = low;
upperBound = high;
scanner = new Scanner(System.in);
}
}
package oop.exceptions;
import java.util.InputMismatchException;
import java.util.Scanner;
public class AgeInput5 {
private static final String DEFAULT_MESSAGE = "Your age:";
private static final int DEFAULT_LOWER_BOUND = 0;
private static final int DEFAULT_UPPER_BOUND = 99;
private int lowerBound;
private int upperBound;
private Scanner scanner;
public AgeInput5() {
init(DEFAULT_LOWER_BOUND, DEFAULT_UPPER_BOUND);
}
public AgeInput5(int low, int high) throws IllegalArgumentException {
if (low > high) {
throw new IllegalArgumentException("Low (" + low + ") was " + "larger than high(" + high + ")");
} else {
init(low, high);
}
}
public int getAge() throws Exception {
return getAge(DEFAULT_MESSAGE);
}
public int getAge(String prompt) throws AgeInputException {
int age;
while (true) {
System.out.print(prompt);
try {
age = scanner.nextInt();
if (age < lowerBound || age > upperBound) {
throw new AgeInputException("Input out of bound", 0, 99, age);
}
return age; // input okay so return the value & exit
} catch (InputMismatchException e) {
scanner.next();
System.out.println("Input is invalid.\n" + "Please enter digits only");
}
}
}
private void init(int low, int high) {
lowerBound = low;
upperBound = high;
scanner = new Scanner(System.in);
}
}
package oop.exceptions;
public class AgeInputException extends Exception {
private static final String DEFAULT_MESSAGE = "Input out of bounds";
private int lowerBound;
private int upperBound;
private int value;
public AgeInputException(int low, int high, int input) {
this(DEFAULT_MESSAGE, low, high, input);
}
public AgeInputException(String msg, int low, int high, int input) {
super(msg);
if (low > high) {
throw new IllegalArgumentException();
}
lowerBound = low;
upperBound = high;
value = input;
}
public int lowerBound() {
return lowerBound;
}
public int upperBound() {
return upperBound;
}
public int value() {
return value;
}
}
package oop.exceptions;
import java.util.Calendar;
import java.util.GregorianCalendar;
import java.util.Scanner;
public class TestAgeInput4 {
public static void main(String[] args) {
GregorianCalendar today;
int age = 0, thisYear, bornYr;
String answer;
Scanner scanner = new Scanner(System.in);
AgeInput4 input = new AgeInput4();
try {
age = input.getAge("How old are you? ");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
today = new GregorianCalendar();
thisYear = today.get(Calendar.YEAR);
bornYr = thisYear - age;
System.out.print("Already had your birthday this year? (Y or N)");
answer = scanner.next();
if (answer.equals("N") || answer.equals("n")) {
bornYr--;
}
System.out.println("\nYou are born in " + bornYr);
}
}
package oop.exceptions;
import java.util.Calendar;
import java.util.GregorianCalendar;
import java.util.Scanner;
public class TestAgeInput5 {
public static void main(String[] args) {
GregorianCalendar today;
int age = 0, thisYear, bornYr;
String answer;
Scanner scanner = new Scanner(System.in);
AgeInput5 input = new AgeInput5();
try {
age = input.getAge("How old are you? ");
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
today = new GregorianCalendar();
thisYear = today.get(Calendar.YEAR);
bornYr = thisYear - age;
System.out.print("Already had your birthday this year? (Y or N)");
answer = scanner.next();
if (answer.equals("N") || answer.equals("n")) {
bornYr--;
}
System.out.println("\nYou are born in " + bornYr);
}
}
Assertion :
package oop.assertions;
public class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
balance = initialBalance;
}
public void deposit(double amount) {
double oldBalance = balance;
balance -= amount;
assert balance > oldBalance;
}
public void withdraw(double amount) {
double oldBalance = balance;
balance -= amount;
assert balance < oldBalance;
}
public double getBalance() {
return balance;
}
public static void main(String[] args) {
BankAccount acct = new BankAccount(200);
acct.deposit(25);
System.out.println("Current Balance: " + acct.getBalance());
}
}